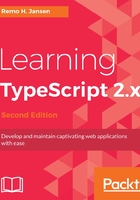
Classes
ECMAScript 6, the next version of JavaScript, adds class-based object-orientation to JavaScript and, since TypeScript includes all the features available in ES6, developers are allowed to use class-based object orientation today, and compile them down to JavaScript that works across all major browsers and platforms, without having to wait for the next version of JavaScript.
Let's take a look at a simple TypeScript class definition example:
class Character { public fullname: string; public constructor(firstname: string, lastname: string) { this.fullname = `${firstname} ${lastname}`; } public greet(name?: string) { if (name) { return `Hi! ${name}! my name is ${this.fullname}`; } else { return `Hi! my name is ${this.fullname}`; } } } let spark = new Character("Jacob","Keyes"); let msg = spark.greet(); console.log(msg); // "Hi! my name is Jacob Keyes" let msg1 = spark.greet("Dr. Halsey"); console.log(msg1); // "Hi! Dr. Halsey! my name is Jacob Keyes"
In the preceding example, we have declared a new class, Character. This class has three members: a property called fullname, a constructor, and a method greet. When we declare a class in TypeScript, all the methods and properties are public by default. We have used the public keyword to be more explicit; being explicit about the accessibility of the class members is recommended but it is not a requirement.
You'll notice that when we refer to one of the members of the class (from within itself), we prepend the this operator. The this operator denotes that it's a member access. In the last lines, we construct an instance of the Character class using a new operator. This calls into the constructor we defined earlier, creating a new object with the Character shape and running the constructor to initialize it.
TypeScript classes are compiled into JavaScript functions in order to achieve compatibility with ECMAScript 3 and ECMAScript 5.