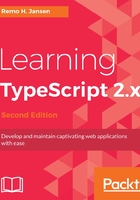
Functions
Just as in JavaScript, TypeScript functions can be created either as a named function or as an anonymous function, which allows us to choose the most appropriate approach for an application, whether we are building a list of functions in an API or a one-off function to hand over to another function:
// named function function greet(name?: string): string { if(name){ return "Hi! " + name; } else { return "Hi!"; } } // anonymous function let greet = function(name?: string): string { if (name) { return "Hi! " + name; } else { return "Hi!"; } }
As we can see in the preceding code snippet, in TypeScript, we can add types to each of the parameters and then to the function itself to add a return type. TypeScript can infer the return type by looking at the return statements, so we can also optionally leave this off in many cases.
There is an alternative syntax for functions that use the => operator after the return type and don't use the function keyword:
let greet = (name: string): string => { if(name){ return "Hi! " + name; } else { return "Hi"; } };
Now that we have learned about this alternative syntax, we can return to the previous example, in which we were assigning an anonymous function to the greet variable. We can now add the type annotations to the greet variable to match the anonymous function signature:
let greet: (name: string) => string = function(name: string):
string { if (name) { return "Hi! " + name; } else { return "Hi!"; } };
Now you know how to add type annotations to force a variable to be a function with a specific signature. The usage of this kind of annotation is really common when we use a callback (functions used as an argument of another function):
function add( a: number, b: number, callback: (result: number) => void ) { callback(a + b); }
In the preceding example, we are declaring a function named add that takes two numbers and a callback as a function. The type annotations will force the callback to return void and take a number as its only argument.