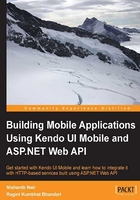
First Kendo UI Mobile application
The best way to learn any new technology or language is to take a deep dive in to it and get your hands dirty. Since this book is all about more and more code, let's start by creating a simple, single page application.
We will follow a progressive enhancement approach starting with basic elements and adding more complexity to the application and, towards the end, we will create a fully functional deployable application.
Note
Kendo UI Mobile supports only WebKit browsers and so it's important to use Chrome or Safari browsers to run the demo code on your desktop.
Now that you have your IDE and emulator ready, perform the following steps to get your first mobile page running:
- Download Kendo UI from the following URL: http://www.kendoui.com/download.aspx. You can either purchase a Kendo UI license or download a free 30-day trial version.
- Create a
root
folder for the application and copy the following mobile-specific files/folders from the Kendo package to this folder:- The
jquery.min.js
andkendo.mobile.min.js
files from thejs
folder to the application root'sjs/kendo
folder - The
kendo.mobile.all.min.css
andimages
folders from thestyles
folder to the application root'sstyles/kendo
folder
- The
- Create an
index.html
file in theroot
folder with the following code in it:<!DOCTYPE HTML> <html> <head> <script src="js/kendo/jquery.min.js"> </script> <script src="js/kendo/kendo.mobile.min.js"></script> <link href="styles/kendo/kendo.mobile.all.min.css" rel="stylesheet" /> </head> <body> <div data-role="view" data-title="Movie Tickets" id="mt-home-main-view" data-layout="mt-main-layout"> Home Page View </div> <div data-role="layout" data-id="mt-main-layout"> <header data-role="header"> <div data-role="navbar"> <span data-role="view-title">Movie Tickets</span> </div> </header> <footer data-role="footer"> <span style="color:#fff"> Footer content goes here </span> </footer> </div> <script> var application = new kendo.mobile.Application(); </script> </body> </html>
Note
Kendo hosts the minified versions of JS and CSS files required for development in a Content Delivery Network (CDN) which can be accessed as follows:
http://cdn.kendostatic.com/
<version>/js/
<filename>.min.js
http://cdn.kendostatic.com/
<version>/styles/
<filename>.min.css
For example:
http://cdn.kendostatic.com/2013.2.716/styles/kendo.mobile.all.min.css
http://cdn.kendostatic.com/2013.2.716/js/kendo.mobile.min.js
- Configure your local web server so that
index.html
can be accessed using a URL; something likehttp://localhost/movietickets/index.html
Note
If you are unfamiliar with how to create a virtual directory in IIS, you can find it here: http://goo.gl/0fUO1. If you are using operating systems other than Windows, please get help from tons of manuals available on the Internet explaining how to host a website locally.
Always remember that this step is required only if you need to test the mobile application using Ripple Emulator. You can always open your website in an HTML5 compatible browser such as Safari or Chrome and see your application running and use their debugging tools too.
Tip
WebKit is an open source browser rendering engine which is used by browsers such as Safari and Chrome. So if you are targeting your app to be bundled using PhoneGap or any other WebKit-based mobile development framework, it's typically safe to test the app using any of the WebKit-based browsers as they share the same rendering engine.
- Open Chrome and navigate to the
index.html
file we created earlier. Click on the Ripple's Enable button and select the device as the latest version of iPhone. If asked for a platform, feel free to select any of the platforms such as PhoneGap, or Mobile Web. We are selecting Apache Cordova/PhoneGap(1.0.0) and iPhone 5 (PhoneGap 2.0.0 version in Ripple may cause the screen to scroll which is not the desired behavior).
Congratulations, your first mobile app using Kendo UI Mobile is up and running!

Tip
If you are planning to bundle your mobile app using a native wrapper such as Cordova/PhoneGap, always bundle the required script files locally and avoid CDNs as this will delay the load time of our application considerably.
Views and Layouts
A view widget represents a page in a Kendo UI Mobile application. All widgets and other HTML elements are added inside a view. Any Kendo UI Mobile app will have one or more views.
A data-role
attribute is used to define what particular mobile widget the HTML element will become once it is rendered.
The attribute definition data-role="view"
defines a view and the data-layout
attribute is used to define which template will act as the layout for our view as shown in our previous example:
<div data-role="view" id="mt-home-main-view" data-layout="mt-main-layout"> Home Page View </div>
A layout is nothing but a master wrapper into which a view will be rendered.
When a view is initialized, all Mobile, Web, and DataViz widgets in the view are initialized in that order.
In the earlier code, we have declared two other roles, header and footer, inside the layout which will render as header and footer of the view respectively.
Tip
An important point to be noted is that since Kendo UI Mobile is designed so that the apps automatically adapt to the native look and feel of the mobile platform on which it runs, the position of the header and footer is reversed for Android and iOS devices due to OS UI design conventions. In iOS, the header is displayed on top and the footer at the bottom of the screen, while the same code in an Android device will display the footer on top and the header at the bottom.
NavBar
The next widget that we encounter is defined as data-role = "navbar"
, which as its definition suggests is the NavBar or the Navigation Bar widget in which we can add a title and/or other widgets. We will see how other widgets are added to the navigation bar soon, but right now we are only adding the title of the application.
Typically NavBar is added in layouts and so depending on the view loaded, the title of the NavBar needs to change. Kendo provides an easy way of doing this.
Just add an HTML element with the attribute data-role="view-title"
inside the NavBar. When the view changes, the value set as the title of the view (using the data-title
attribute of the view) will be automatically displayed in the NavBar inside the element decorated with the view-title
role:
<!-- navbar definition --> <div data-role="layout" data-id="my-layout"> <div data-role="navbar"> <span data-role="view-title">My View Title</span> </div> </div> <!-- view 1 --> <div data-role="view" data-layout="my-layout"data-title="View 1 Title"></div> <!-- view 2 --> <div data-role="view" data-layout="my-layout"data-title="View 2 Title"></div>
Application initialization
The HTML code that we discussed earlier contains definitions of widgets only and to bring them to life, we need to initialize our app as a Kendo UI Mobile app. This is done using a small magical piece of code:
var application = new kendo.mobile.Application();
This piece of code initializes your Kendo UI Mobile application and gives life to all the widgets and it needs to be added just before the </body>
tag.
The application initialization script is very powerful and can be extended to set a lot of global properties of the application which we will explore later in the chapter.
Tip
All Kendo UI Web widgets are highly touch optimized and designed to run on mobile devices too. So you can always use a Web widget inside a mobile application if the need arises.