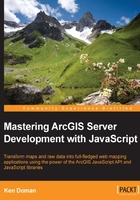
Finding the ArcGIS JavaScript API documentation
You can find the documentation for the ArcGIS API for JavaScript by visiting https://developers.arcgis.com/javascript/jsapi/. There, you'll find information on the latest version of the API, a list of changes since the previous version, and related documents to help you create apps. The organization is logical, and information is relatively easy to find with their layout.
The documentation layout
The documentation for each element in the ArcGIS API is laid out in an organized fashion. Links to the API components are on the left-hand side. The documentation for each element defaults to the AMD style, but provides links to the older legacy development style. With the AMD style, the top of the page shows how to load the module into your code. Following that is a description of the module, a link to samples that use the module, and a class hierarchy diagram showing the modules the current module inherits.
If the module requires a JavaScript object to be constructed, the documentation provides information on what is needed to call the module's constructor. The documentation provides details on the parameters required to create the objects, including parameter names, object types, whether they're optional or not, and what they do.
Following any constructor information, the documentation provides data on the CSS class attributes for anything that the module may show on the map, as well as any HTML5 data-* attributes the module might employ. The CSS classes help you to modify the appearance of widgets and visual elements on the module, by giving you a class hook to modify with your custom styling. The data-* attributes give other JavaScript scripts access to information about your widget, without having to load the whole widget into memory. For instance, by clicking on a map graphic while using another library, you could access the geometry's type by looking at the element's data-geometry-type attribute.
When an ArcGIS API object is created using the constructor, it will have certain properties, or variable parameters assigned to the object. Some properties are set when the object is created. Other properties are set as the object is modified, or certain methods are run. The API documentation lists object properties that are actively maintained, and will not be removed without sufficient notice of depreciation.
Tip
If you use web developer tools in your browser, such as Firebug for Firefox, or Chrome Developer tools, you might find that these ArcGIS API objects have more properties than are listed in the documentation. Many properties considered private will have one or two underscore (_
) characters before the name. If the properties and methods are not listed in the documentation, they may not be there when the next version of the API is released. Don't count on undocumented properties and methods in your code.
Besides certain properties, objects created with the ArcGIS API modules will probably contain methods, which are JavaScript functions assigned to the object, often using other parts of the object they're assigned to. The documentation will list public methods attached to the object, as well as what parameters they accept, what values they return, and a description of what task or function they perform.
Many of the modules have documented events, where the module emits a notification that something has happened. These are similar to a HTML button's onClick
event, or a browser's onLoad
event. In older versions of the API, events were listened to using dojo.connect
. But as the library has matured, Dojo is depreciating the connect function and replacing it with the dojo/on library (http://dojotoolkit.org/reference-guide/1.10/dojo/on.html). The dojo/on
module actually delivers a function.
With the dojo/on function, you attach events by calling it with the following parameters: the HTML DOM elements or Dojo widgets you want to listen to, the name of the event as a string, and a function that will be called when the event occurs. If you want to stop the event, you need a variable to accept the returned value of the dojo/on call, ahead of time. When you're ready to stop listening, call that return variable's remove()
method. Some modules contain their own on()
method, which implements the dojo/on
module for event handling. Here's an example code snippet using the dojo/on
module:
require(["dojo/on", "dojo/dom", "dojo/domReady!"], function (on, dom) { var soupMe = on(dom.byId("deliver-soup"), "click", function () { alert("Here's your soup. NEXT!"); }); on(dom.byId("no-more-soup"), "click", function () { alert("NO SOUP FOR YOU!"); soupMe.remove(); }); });
Now that we know what to expect when we're reading the documentation, let's look at the different tools available in the ArcGIS API for JavaScript. Since ArcGIS applications work with geographic data, we'll start off by looking at the map.