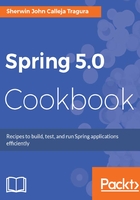
上QQ阅读APP看书,第一时间看更新
How to do it...
Let us illustrate the eager and lazy loading of beans in a context definition using these steps:
- In the case of the XML-based ApplicationContext, eager loading means all the beans in the definition will be loaded and initialized aggressively in the heap memory during start-up (for example, pre-instantiating). To opt for this type of initialization, each <bean> has an attribute lazy-init, which must be set to false. Open ch02-beans.xml in the project ch02-xml and add the following bean:
<bean id="empRec6" class="org.packt.starter.ioc.model.Employee" lazy-init="false"> <constructor-arg><value>Diego</value></constructor-arg> <constructor-arg><value>Silang</value></constructor-arg> <constructor-arg> <value>December 16, 1965</value>
</constructor-arg> <constructor-arg><value>55</value></constructor-arg> <constructor-arg><value>87000</value></constructor-arg> <constructor-arg><value>Guitarist</value></constructor-arg> <constructor-arg><ref bean="dept4"></ref></constructor-arg> </bean>
By default, all Spring beans load in eager mode since lazy-init="auto" also means lazy-init="false". Also, all Singleton beans use eager loading automatically.
- In the case of the JavaConfig approach, by default @Bean loads aggressively and we do not have a special annotation or metadata for eager initialization.
- The other option of bean loading happens only during the fetching stage (for example, the use of the getBean() method) and this is called lazy loading. In the XML-based container, we set the lazy-init attribute of a <bean> to true to implement lazy bean loading. Using the same class models in Step 1, apply the necessary changes as follows:
<bean id="empRec6" class="org.packt.starter.ioc.model.Employee" lazy-init="true"> // refer to sources </bean>
All Prototype beans use the lazy loading mode.
- For JavaConfig, Spring has a method-level annotation @Lazy, which can be applied to @Bean objects to perform lazy loading:
@Lazy @Bean public Employee empRec4(Department dept2){ Employee empRec4 = new Employee("Diego","Silang", new Date(65,11, 15), 55, 85000.00, "guitarist", dept4()); return empRec4; }
Setting @Lazy(value=false) makes the loading mode eager.