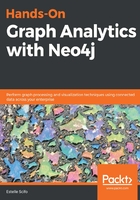
Updating and deleting nodes and relationships
Creating objects is not sufficient for a database to be useful. It also needs to be able to do the following:
- Update existing objects with new information
- Delete objects that are no longer relevant
- Read data from the database
This section deals with the first two bullet points, while the last one will be covered in the following section.
Updating objects
There is no UPDATE keyword with Cypher. To update an object, node, or relationship, we'll use the SET statement only.
Updating an existing property or creating a new one
If you want to update an existing property or add a new one, it's as simple as the following:
MATCH (n {id: 1})
SET n.name = "Node 1"
RETURN n
The RETURN statement is not mandatory, but it is a way to check the query went well, for instance, checking the Table tab of the result cell:
{
"name": "Node 1",
"id": 1
}
Updating all properties of the node
If we want to update all properties of the node, there is a practical shortcut:
MATCH (n {id: 1})
SET n = {id: 1, name: "My name", age: 30, address: "Earth, Universe"}
RETURN n
This leads to the following result:
{
"name": "My name",
"address": "Earth, Universe",
"id": 1,
"age": 30
}
In some cases, it might be painful to repeat existing properties to be sure not to erase the id, for instance. In that case, the += syntax is the way to go:
MATCH (n {id: 1})
SET n += {gender: "F", name: "Another name"}
RETURN n
This again works as expected, adding the gender property and updating the value of the name field:
{
"name": "Another name",
"address": "Earth, Universe",
"id": 1,
"gender": "F",
"age": 30
}
Updating node labels
On top of adding, updating, and deleting properties, we can do the same with node labels. If you need to add a label to an existing node, you can use the following syntax:
MATCH (n {id: 1})
SET n:AnotherLabel
RETURN labels(n)
Here, again, the RETURN statement is just there to make sure everything went well. The result is as follows:
["Label", "AnotherLabel"]
On the contrary, if you mistakenly set a label to a node, you can REMOVE it:
MATCH (n {id: 1})
REMOVE n:AnotherLabel
RETURN labels(n)
And we are back to the situation where the node with id:1 has a single label called Label.
Deleting a node property
We briefly talked about NULL values in the previous chapter. In Neo4j, NULL values are not saved in the properties list. An absence of a property means it's null. So, deleting a property is as simple as setting it to a NULL value:
MATCH (n {id: 1})
SET n.age = NULL
RETURN n
Here's the result:
{
"name": "Another name",
"address": "Earth, Universe",
"id": 1,
"gender": "F" }
The other solution is to use the REMOVE keyword:
MATCH (n {id: 1})
REMOVE n.address
RETURN n
The result would be as follows:
{
"gender": "F",
"name": "Another name",
"id": 1
}
If you want to remove all properties from the node, you will have to assign it an empty map like so:
MATCH (n {id: 2})
SET n = {}
RETURN n
Deleting objects
To delete an object, we will use the DELETE statement:
- For a relationship:
MATCH ()-[r:REL_TYPE {id: 1}]-()
DELETE r
- For a node:
MATCH (n {id: 1})
DELETE n
Deleting a node requires it to be detached from any relationship (Neo4j can't contain a relationship with a NULL extremum).
If you try to delete a node that is still involved in a relationship, you will receive a Neo.ClientError.Schema.ConstraintValidationFailed error, with the following message:
Cannot delete node<41>, because it still has relationships. To delete this node, you must first delete its relationships.
We need to first delete the relationship, and then the node in this way:
MATCH (n {id:1})-[r:REL_TYPE]-()
DELETE r, n
But here again, Cypher provides a practical shortcut for this – DETACH DELETE – which will perform the preceding operation:
MATCH (n {id: 1})
DETACH DELETE n
You now have all the tools to in hand to create, update, delete, and read simple patterns from Neo4j. In the next section, we will focus on the pattern matching technique, to read data from Neo4j in the most effective way.