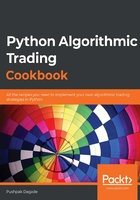
Modifying datetime objects
Often, you may want to modify existing datetime objects to represent a different date and time. This recipe includes code to demonstrate this.
How to do it…
Follow these steps to execute this recipe:
- Import the necessary modules from the Python standard library:
>>> from datetime import datetime
- Fetch the current timestamp. Assign it to dt1 and print it:
>>> dt1 = datetime.now()
>>> print(dt1)
We get the following output. Your output would differ:
2020-08-12 20:55:46.753899
- Create a new datetime object by replacing the year, month, and day attributes of dt1. Assign it to dt2 and print it :
>>> dt2 = dt1.replace(year=2021, month=1, day=1)
>>> print(f'A timestamp from 1st January 2021: {dt2}')
We get the following output. Your output would differ:
A timestamp from 1st January 2021: 2021-01-01 20:55:46.753899
- Create a new datetime object by specifying all the attributes directly. Assign it to dt3 and print it:
>>> dt3 = datetime(year=2021,
month=1,
day=1,
hour=dt1.hour,
minute=dt1.minute,
second=dt1.second,
microsecond=dt1.microsecond,
tzinfo=dt1.tzinfo)
print(f'A timestamp from 1st January 2021: {dt3}')
We get the following output. Your output would differ:
A timestamp from 1st January 2021: 2021-01-01 20:55:46.753899
- Compare dt2 and dt3:
>>> dt2 == dt3
We get the following output.
True
How it works...
In step 1, you import the datetime class from the datetime module. In step 2, you fetch the current timestamp using the now() method of datetime and assign it to a new attribute, dt1. To get a modified timestamp from an existing datetime object, you can use the replace() method. In step 3, you create a new datetime object dt2, from dt1, by calling the replace() method. You specify the attributes to be modified, which are year, month, and day. The remaining attributes remain as it is, which are an hour, minute, second, microsecond, and timezone. You can confirm this by comparing the outputs of step 2 and step 3. In step 4, you create another datetime object, dt3. This time you call the datetime constructor directly. You pass all the attributes to the constructor such that the timestamp created is the same as dt2. In step 5, you confirm that dt2 and dt3 hold exactly the same timestamp by using the == operator, which returns True.