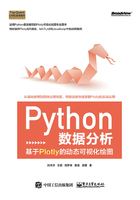
2.3 散点图
2.3.1 基本案例
线形图又称为曲线图,是最常用的图形类型。与传统的绘图软件不同,Plotly没有独立的线形图函数,而是把线形图与散点图全部用Scatter函数实现。
下面的代码(见文件scatter_basic_demo.py)是基本散点图的绘制方法,包括线形图与散点图两种图形的混合。
import plotly as py import plotly.graph_objs as go # ----------pre def pyplt = py.offline.plot # ----------code # Create random data with numpy import numpy as np N = 100 random_x = np.linspace(0, 1, N) random_y0 = np.random.randn(N)+5 random_y1 = np.random.randn(N) random_y2 = np.random.randn(N)-5 # Create traces trace0 = go.Scatter( x = random_x, y = random_y0, mode = 'markers', # 纯散点的绘图 name = 'markers' # 曲线名称 ) trace1 = go.Scatter( x = random_x, y = random_y1, mode = 'lines+markers', # “散点+线”的绘图 name = 'lines+markers' ) trace2 = go.Scatter( x = random_x, y = random_y2, mode = 'lines', # 线的绘图 name = 'lines' ) data = [trace0, trace1, trace2] pyplt(data, filename='tmp\scatter_basic_demo.html')
代码运行结果如图2-4所示。

图2-4 代码运行结果
查看案例源码可以看出,markers、lines和lines+markers三个图形的输出格式不同,是因为Scatter函数中的mode参数不同:
mode = 'markers', #xtr1,散点图 mode = 'lines', #xtr2,线形图, mode = 'lines + markers', #xtr3,线形图+散点图组合
2.3.2 样式设置
下面的代码(见文件scatter_style.py)用来设置点的大小和颜色,以及线条的大小和颜色。
import plotly as py import plotly.graph_objs as go import numpy as np # ----------pre def pyplt = py.offline.plot # ----------code N = 500 x = np.random.randn(N) trace0 = go.Scatter( x = np.random.randn(N), y = np.random.randn(N)+2, name = 'Above', mode = 'markers+lines', marker = dict( size = 10, # 设置点的宽度 color = 'rgba(152, 0, 0, .8)', # 设置点的颜色 line = dict( width = 2, # 设置线条的宽度 color = 'rgb(0, 0, 0)' # 设置线条的颜色 ) ) ) trace1 = go.Scatter( x = np.random.randn(N), y = np.random.randn(N) -2, name = 'Below', mode = 'markers', marker = dict( size = 10, color = 'rgba(255, 182, 193, .9)', line = dict( width = 2, ) ) ) data = [trace0, trace1] layout = dict(title = 'Styled Scatter', yaxis = dict(zeroline = True), # 显示y轴的0刻度线 xaxis = dict(zeroline = False) # 不显示x轴的0刻度线 ) fig = dict(data=data, layout=layout) pyplt(fig, filename='tmp\scatter_style.html')
代码运行结果如图2-5所示。

图2-5 代码运行结果
在这个案例中,重要的是marker参数的设置:
marker = dict( size = 10, # 设置点的宽度 color = 'rgba(152, 0, 0, .8)', # 设置点的颜色 line = dict( width = 2, # 设置线条的宽度 color = 'rgb(0, 0, 0)' # 设置线条的颜色 ) )
这里size设置的是点的宽度,width设置的是线条的宽度,第一个color设置的是点的颜色,第二个color设置的是线条的颜色,读者可以对这些参数进行修改,实际验证一下。
需要注意的是,Plotly中的这些参数在其他绘图函数中可以重复使用,这也是Plotly人性化的地方。
2.3.3 应用案例
本案例的文件名是scatter_apply.py,讲解Scatter函数的使用方法,绘制曲线图与散点图,以及曲线图与散点图的组合图,代码如下。
# -*- coding: utf-8-*- import pandas as pd import pandas as pd import plotly as py import plotly.graph_objs as pygo # ----------pre def pd.set_option('display.width', 450) pyplt = py.offline.plot # ----------code df = pd.read_csv('dat/tk01_m15.csv') df9 = df[:10]; print(df9) # idx = df9['xtim'] xd0 = (df9['close'] -27) * 50 df2 = df9 df2['xd1'] = xd0-10 df2['xd2'] = xd0 df2['xd3'] = xd0 + 10 print('xd2\n', df2); # -------- xtr1 = pygo.Scatter( x=idx, y=df2['xd1'], mode='markers', # xtr1,散点图 name='xtr1-markers', ) xtr2 = pygo.Scatter( x=idx, y=df2['xd2'], mode='lines', # xtr2,曲线图 name='xtr2-lines', ) xtr3 = pygo.Scatter( x=idx, y=df2['xd3'], mode='markers+lines', # xtr3,曲线图+散点图 name='xtr3-markers+lines', ) xdat = pygo.Data([xtr1, xtr2, xtr3]) layout = pygo.Layout( title=’收盘价--15分钟分时数据’, xaxis=pygo.XAxis(tickangle=-15), ) fig = pygo.Figure(data=xdat, layout=layout, filename=r'tmp\ scatter_apply.html') pyplt(fig) # print('ok')
代码运行结果如图2-6所示。

图2-6 代码运行结果
2.3.4 参数解读
使用Scatter函数可以绘制线形图与散点图,主要参数如下。
●connectgaps:布尔变量,用于连接缺失数据。
●dx、dy:x、y坐标轴的步进值,默认值是1。
●error_x、error_y:x、y出错信息。
●fillcolor:填充颜色。
●fill:填充模式,包括格式、颜色等。
●hoverinfo:当用户与图形互动时,鼠标指针显示的参数,包括x、y、z坐标数据,以及text(文字信息)、name(图形名称)等参数的组合,可使用+、all、none和skip(忽略)作为组合连接符号,默认是all(全部消失)。
●hoveron:当用户与图形互动时,鼠标指针显示的模式,包括points(点图)、fills(填充图)和points+fills(点图+填充图)三种模式。
●ids:在动画图表中,数据点和图形key键的列表参数。
●legendgroup:图例参数,默认是空字符串。
●line:线条参数,包括线条宽度、颜色、格式。
●marker:数据节点参数,包括大小、颜色、格式等。
●mode:图形格式,包括lines(线形图)、markers(散点图)和text(文本),使用+或none等符号进行模式组合。
●name:名称参数。
●opacity:透明度参数,范围是0~1。
●rsrc、xsrc、ysrc、tsrc、idssrc、textsrc、textpositionsrc:字符串源数组列表,可作为Plotly网格标识符,用于设置特殊图表所需的r参数、x参数、y参数、t参数、ids参数、text(文本)参数和textposition(文本位置)参数。
●r、t:仅用于极坐标图,r用于设置径向坐标(半径),t用于设置角坐标。
●showlegend:布尔变量,用于切换图标显示。
●stream:数据流,用于实时显示数据图表。
●textfont:文本字体参数,包括字体名称、颜色、大小等。
●textposition:“文本”元素的位置参数,包括top left(左上)、top center(中上)、top right(右上)、middle left(左中)、middle center(中心)、middle right(右中)、bottom left(左下)、bottom center(中下)、bottom right(右下)模式,默认是middle center(中心)模式。
●text:文本数据,设置与每个“(x, y)对”关联的文本元素和数组列表格式,默认是空字符串。
●type:数据显示模式,包括constant(常数)、percent(百分比)、sqrt(平方根)、array(数组)模式。
●x0、y0:坐标轴起点坐标。
●xaxis, yaxis:x、y坐标参数。
●xcalendar、ycalendar:坐标时间参数的格式,默认是公历(gregorian),支持gregorian、chinese、coptic、discworld、ethiopian、hebrew、islamic、julian、mayan、nanakshahi、nepali、persian、jalali、taiwan、thai和ummalqura格式。
●x, y:设置x、y轴的坐标数据。